pythonのフレームワークFlaskを使ってWEBアプリを作る。
PythonのフレームワークFlaskでWEBアプリを作成する
flaskは軽量なWEBアプリフレームワークです。
WEBアプリ作成に必要なものは主に3つです。
- Flask
- pythonフレームワーク
- pubchempy
- 化合物名からSMILESを取得
- rdkit
- SMILESから画像を生成
まずはpythonのスクリプトを作成する
アプリケーションのコードを作成します。
from flask import Flask, render_template, request
import pubchempy as pcp
from rdkit import Chem
from rdkit.Chem import Draw
from io import BytesIO
import base64
app = Flask(__name__)
@app.route("/")
def home():
return render_template('index.html')
@app.route("/submit", methods=['POST'])
def submit():
if request.method == 'POST':
compound_name = request.form['compound_name']
compound = pcp.get_compounds(compound_name, 'name')[0]
smiles = compound.canonical_smiles
mol = Chem.MolFromSmiles(smiles)
img = Draw.MolToImage(mol)
img_buffer = BytesIO()
img.save(img_buffer, format='PNG')
img_buffer.seek(0)
img_base64 = base64.b64encode(img_buffer.getvalue()).decode('utf-8')
return render_template('result.html', compound_name=compound_name, smiles=smiles, img_base64=img_base64)
if __name__ == '__main__':
app.run()
・ app = Flask(__name__)
→Flaskのインスタンスを作成
・ def home():
→127.0.0.1:5000/にアクセスしてきた時に実行される関数
return render_template(‘index.html’) → index.html をレンダリングして返す
・ def submit():
→/submit にPOSTリクエストが送られたときに実行される関数
・ compound_name = request.form[‘compound_name’] は、POSTリクエストで送られたフォームの値(name=compound_name)を取得してcompound_nameに代入
coompound = pcp.get_compounds(compound_name, ‘name’)[0] →PubChemから化合物を検索する
・ img = Draw.MolToImage(mol) →画像を作成する
・ img_buffer = BytesIO() →画像をバイト列に変換するためバッファを作成する
・ img.save(img_buffer, format=’PNG’) →バッファに画像を保存する
・ img_buffer.seek(0) →バッファの先頭にポインタを移動する
・ img_base64 = base64.b64encode(img_buffer.getvalue()).decode(‘utf-8’) →バッファの内容をBase64エンコードする
表示用のhtmlファイルを作成
トップページのindex.htmlと結果を返却するresult.htmlを作成します。
<!DOCTYPE html>
<html>
<head>
<title>generate chemical structure image and SMILES</title>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Bootstrap demo</title>
<link href="https://cdn.jsdelivr.net/npm/bootstrap@5.2.0/dist/css/bootstrap.min.css" rel="stylesheet" integrity="sha384-gH2yIJqKdNHPEq0n4Mqa/HGKIhSkIHeL5AyhkYV8i59U5AR6csBvApHHNl/vI1Bx" crossorigin="anonymous">
</head>
<body>
<h2 style="text-align:center">化学物質の名前を英語で入力してください</h2>
<form action="/submit" method="post">
<div class="container">
<input type="text" class="form-control" name="compound_name" id="compoond_name">
<div class="container d-flex justify-content-center">
<button type="submit" value="Submit" class="btn btn-primary">送信</button>
<script src="js/bootstrap.bundle.min.js"></script>
</div>
</div>
</form>
</body>
</html>
次にresult.htmlを作成します
<!DOCTYPE html>
<html>
<head>
<meta charset="utf-8">
<meta name="viewport" content="width=device-width, initial-scale=1">
<title>Result</title>
<link rel="stylesheet" href="https://cdnjs.cloudflare.com/ajax/libs/bootstrap/5.1.3/css/bootstrap.min.css">
</head>
<body>
<h1>{{ compound_name }}</h1>
<p>SMILES: {{ smiles }}</p>
<img src="data:image/png;base64,{{ img_base64 }}" alt="chemical structure">
<br><a href="/" class="btn btn-secondary">Back</a>
</body>
</html>
装飾のためのbootstrapを入れてますが、なくても問題ありません。
入れないとコードはもっとシンプルになります。
実行
以下のコマンドを実行します
# python app.py
サーバが立ち上がるので、http://127.0.0.1:5000/ にアクセスします。
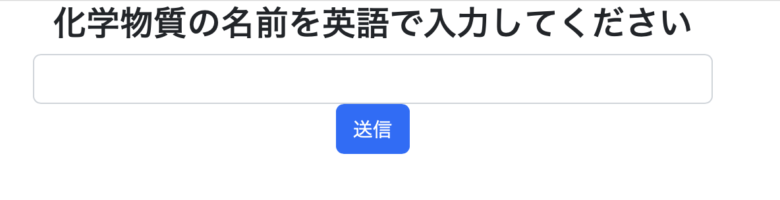
benzeneと入力して送信すると下記の画像が表示されます。
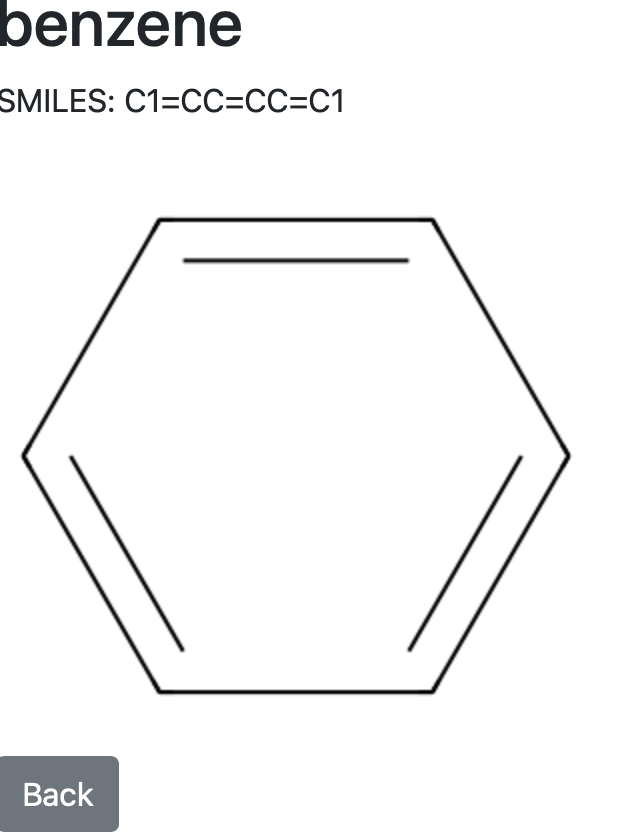
flaskは軽量で完結なフレームワークなのですぐにWEBアプリを作成できて便利です。